Advent of Code 2024: Day 1
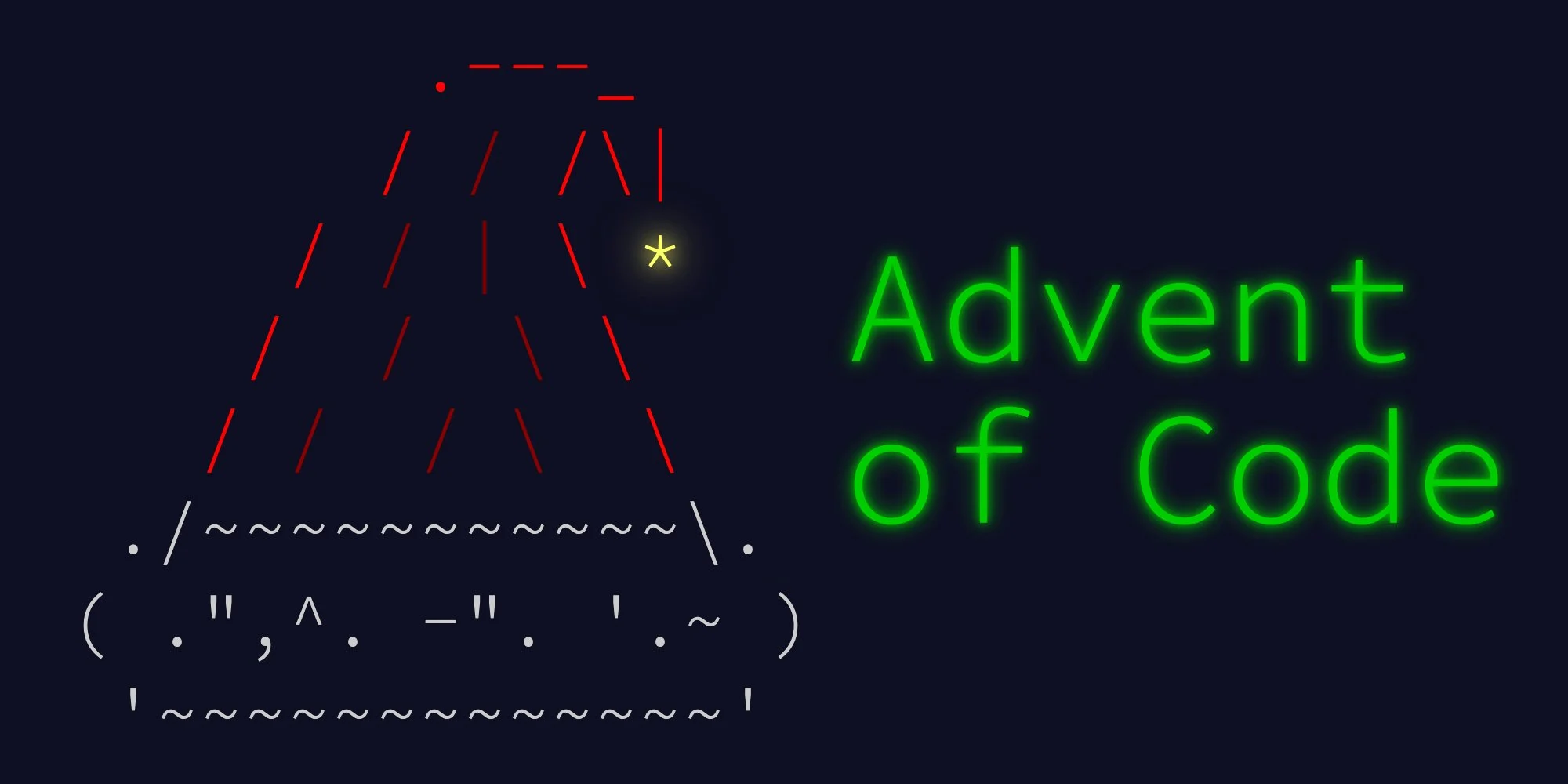
And off we go with a new year of Advent of code! 🥳 🎄
After a several years using Java and (mostly) Kotlin, along with some Python, I've decided to solve this year's tasks using TypeScript - part because it's the language I use for work, so I figured it's a good way to practise, part because I wanted some change and new challenges.
The first day is easy, as usual. The input consists of two lists like this:
3 4
4 3
2 5
1 3
3 9
3 3
The goal is to pair the smallest number of the left list with the smallest of the right list, then the second-smallest numbers, etc. Fundamentally we need to sort both in an ascending order. After we established the pairs, we need to compute the difference, and sum everything up.
For example, if we sort the test input above and we calculate the differences:
1 3 => 2
2 3 => 1
3 3 => 0
3 4 => 1
3 5 => 2
4 9 => 5
resulting in a total of 11.
My solution for the first task is also pretty simple:
function calcDistanceChecksum(leftList: number[], rightList: number[]): number {
let distanceChecksum = 0;
leftList.forEach((num, index) => {
// Not convinced that the absolute value is actually needed
distanceChecksum += Math.abs(num - rightList[index]);
});
return distanceChecksum;
}
Part 2 goes like this: for each number on the left list, we need to find how many times such number occurs in the right list. Based on this, we can compute the similarity score consisting of the number multiplied by the occurrences count.
Using again the same example:
3
occurs three times on the right →9
4
occurs once →4
2
does not occur →0
1
does not occur →0
Finally we count the score of 3 three times, since it appears multiple times in the left list, bringing to a total score of 31.
Again, the function is fairly straightforward:
function calcSimilarityScore(leftList: number[], rightList: number[]): number {
let totalSimilarityScore = 0;
leftList.forEach((num) => {
const occurrences = rightList.filter(
(rightNum) => rightNum === num
).length;
totalSimilarityScore += num * occurrences;
});
return totalSimilarityScore;
}
And that's the first two stars in the bag! ⭐⭐